Stat bars
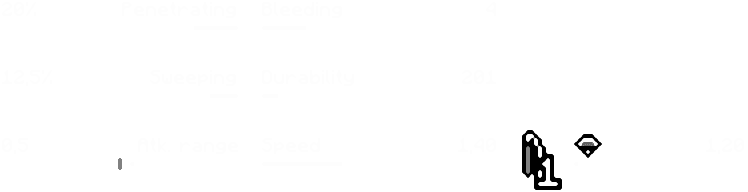
Stat bars are used to display different stats on an item, like effects, attributes or durability. They can either be registered programmatically during initialization of an addon mod, or defined in a resourcepack.
Programmatically defined stat bars can be registered during the FMLClientSetupEvent
event by calling WorkbenchStatsGui.addBar(statBar)
to make the stat bar show up in the workbench, and HoloStatsGui.addBar(statBar)
to make it show up in the holosphere.
Short example:
static final ItemEffect ignitingEffect = ItemEffect.get("igniting");
/* ... */
@SubscribeEvent
@OnlyIn(Dist.CLIENT)
public void clientSetup(FMLClientSetupEvent event) {
var statGetter = new StatGetterEffectLevel(ignitingEffect, 1);
GuiStatBar statBar = new GuiStatBar(0, 0, StatsHelper.barLength,
"tetra.stats.igniting", 0, 10, false, false, false,
statGetter, LabelGetterBasic.integerLabel,
new TooltipGetterInteger("tetra.stats.igniting.tooltip", statGetter)
);
WorkbenchStatsGui.addBar(statBar);
HoloStatsGui.addBar(statBar);
}
Remember to register the event handler!
Data driven stat bars
Stat bars can be defined in JSON files within resources/tetra/stat_bars/
in a resourcepack (not a datapack!).
Stat Bar
Defines how a statbar displays, including labels, formatting, tooltip and possibly bar indicators.
Format:There's only one type registered in vanilla tetra, but this can be set if some other mod has registered other statbar types.
The minimum value for the stat bar, sets the lower bound for the actual bar.
The maximum value for the stat bar, sets the upper bound for the actual bar.
Defines which UIs to show this stat bar in.
If set to true, registers a stat sorter with the same label, stat and format as this stat bar.
{
"type": "tetra:default",
"key": "tetra.stats.attack_damage",
"contexts": [
"tetra:workbench"
],
"min": 0,
"max": 40,
"stat": {
"type": "tetra:sum",
"stats": [
{
"type": "tetra:attribute",
"attribute": "generic.attack_damage"
},
{
"type": "tetra:sum",
"stats": [
{
"type": "tetra:multiply",
"stats": [
{
"type": "tetra:enchantment",
"enchantment": "minecraft:sharpness"
}
],
"factor": 0.5
}
],
"offset": 0.5
}
]
},
"label": {
"type": "tetra:basic",
"format": "double_decimal",
"diff_format": "double_decimal"
},
"tooltip": {
"type": "tetra:default",
"key": "tetra.stats.attack_damage.tooltip",
"stats": [
{
"type": "tetra:sum",
"stats": [
{
"type": "tetra:attribute",
"attribute": "generic.attack_damage"
},
{
"type": "tetra:sum",
"stats": [
{
"type": "tetra:multiply",
"stats": [
{
"type": "tetra:enchantment",
"enchantment": "minecraft:sharpness"
}
],
"factor": 0.5
}
],
"offset": 0.5
}
],
"attribute": "generic.attack_damage"
}
],
"formatters": [
{
"type": "tetra:basic",
"format": "double_decimal"
}
]
},
"indicators": [
"tetra:attack_damage/shared",
"tetra:attack_damage/normalized"
]
}